Spring boot
Bank App 만들기 - 간단한 유틸클래스 만들기
햄발자
2024. 9. 27. 15:36
작업 목표
1. 시간, 금액에 대한 포멧 적용하기
2. account/detail.jsp 파일 코드 수정 하기
1. 시간, 금액에 대한 포멧 적용하기
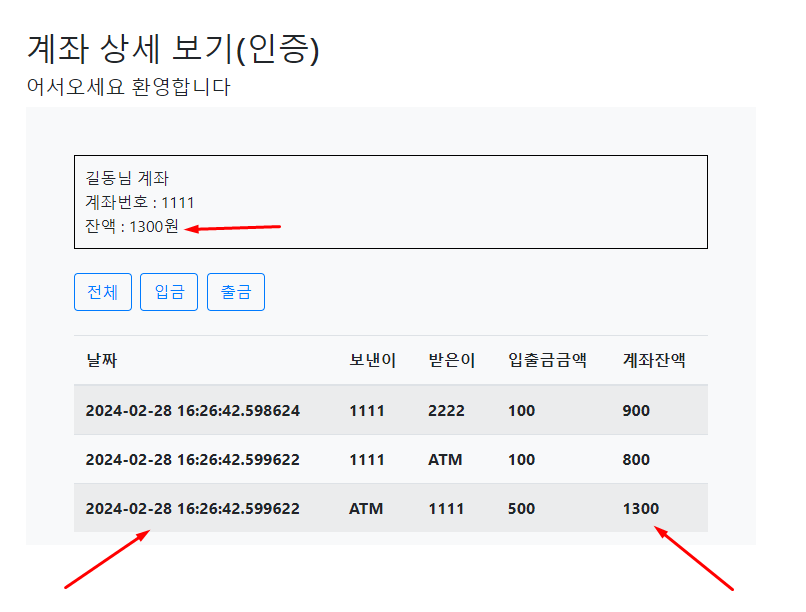
ValueFormatter.java
더보기
닫기
ValueFormatter.java
package com.tenco.bank.utils;
import java.sql.Timestamp;
import java.text.DecimalFormat;
import java.text.SimpleDateFormat;
public abstract class ValueFormatter {
// 시간 포맷
public String timestampToString(Timestamp timestamp) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-DD HH:mm:ss");
return sdf.format(timestamp);
}
public String formatKoreanWon(Long amount) {
DecimalFormat df = new DecimalFormat("#,###");
String formatNumber = df.format(amount);
return formatNumber + "원";
}
}
Account.java
더보기
닫기
Account.java
package com.tenco.bank.repository.model;
import java.sql.Timestamp;
import org.springframework.http.HttpStatus;
import com.tenco.bank.handler.exception.DataDeliveryException;
import com.tenco.bank.utils.Define;
import com.tenco.bank.utils.ValueFormatter;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.ToString;
@Data
@NoArgsConstructor
@AllArgsConstructor
@Builder
@ToString
public class Account extends ValueFormatter {
private Integer id;
private String number;
private String password;
private Long balance;
private Integer userId;
private Timestamp createdAt;
// 출금 기능
public void withdraw(Long amount) {
// 방어적 코드
this.balance -= amount;
}
// 입금 기능
public void deposit(Long amount) {
this.balance += amount;
}
// 패스워드 체크 기능
public void checkPassword(String password) {
// f == f 일때 ---> true
if(this.password.equals(password) == false ) {
// 패스워드 틀렸을 시 (작동)
throw new DataDeliveryException(Define.FAIL_ACCOUNT_PASSWROD, HttpStatus.BAD_REQUEST);
}
}
// 잔액 여부 확인 기능 - checkBalance
public void checkBalance(Long amount) {
//
if(this.balance < amount) {
// 잔액 부족 (출금 잔액이 부족)
throw new DataDeliveryException(Define.LACK_Of_BALANCE, HttpStatus.BAD_REQUEST);
}
}
// 계좌 소유자 확인 기능 (세션 확인 해서?) - checkOwner
public void checkOwner(Integer principalId) {
//
if(this.userId != principalId) {
// 계좌 소유자가 아니다.
throw new DataDeliveryException(Define.NOT_ACCOUNT_OWNER, HttpStatus.BAD_REQUEST);
}
}
}
HistoryRepository.java
더보기
닫기
HistoryRepository.java
package com.tenco.bank.repository.interfaces;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import com.tenco.bank.repository.model.History;
import com.tenco.bank.repository.model.HistoryAccount;
// HistoryRepository, history.xml 파일을 매칭 시킨다.
@Mapper
public interface HistoryRepository {
public int insert(History history);
public int updateById(History history);
public int deleteById(Integer id);
// 거래내역 조회
public History findById(Integer id);
public List<History> findAll();
// 코드 추가 예정 - 모델을 반드시 1:1 엔티티에 매핑을 시킬 필요는 없다.
// 조인 쿼리, 서브쿼리, 동적쿼리, type=all, de.. , accountId
public List<HistoryAccount> findByAccountIdAndTypeOfHistory(@Param("type") String type, @Param("accountId") Integer accountId);
}
deposit.jsp
더보기
닫기
deposit.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!-- header.jsp -->
<%@ include file="/WEB-INF/view/layout/header.jsp"%>
<!-- start of content.jsp(xxx.jsp) -->
<div class="col-sm-8">
<h2>입금 요청(인증)</h2>
<h5>Bank App에 오신걸 환영합니다</h5>
<form action="/account/deposit" method="post">
<div class="form-group">
<label for="amount">입금 금액:</label>
<input type="number" class="form-control" placeholder="Enter amount"
id="amount" name="amount" value="1000">
</div>
<div class="form-group">
<label for="dAccountNumber">입금 계좌 번호:</label>
<input type="text" class="form-control" placeholder="Enter account number"
id="dAccountNumber" name="dAccountNumber" value="1002-1234">
</div>
<div class="text-right">
<button type="submit" class="btn btn-primary">입금</button>
</div>
</form>
</div>
<!-- end of col-sm-8 -->
</div>
</div>
<!-- end of content.jsp(xxx.jsp) -->
<!-- footer.jsp -->
<%@ include file="/WEB-INF/view/layout/footer.jsp"%>
detail.jsp
더보기
닫기
detail.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!-- header.jsp -->
<%@ include file="/WEB-INF/view/layout/header.jsp"%>
<!-- start of content.jsp(xxx.jsp) -->
<div class="col-sm-8">
<h2>계좌 상세 보기(인증)</h2>
<h5>Bank App에 오신걸 환영합니다.</h5>
<div class="bg-light p-md-5 p-75">
<div class="user--box">
${principal.username}님 계좌 <br> 계좌번호 : ${account.number} <br> 잔액 : ${account.formatKoreanWon(account.balance)}
</div>
<br>
<div>
<a href="/account/detail/${account.id}?type=all" class="btn btn-outline-primary" >전체</a>
<a href="/account/detail/${account.id}?type=deposit" class="btn btn-outline-primary" >입금</a>
<a href="/account/detail/${account.id}?type=withdrawal" class="btn btn-outline-primary" >출금</a>
</div>
<br>
<table class="table table-striped">
<thead>
<tr>
<th>날짜</th>
<th>보낸이</th>
<th>받은이</th>
<th>입출금 금액</th>
<th>계좌잔액</th>
</tr>
</thead>
<tbody>
<c:forEach var='historyAccount' items = "${historyList}">
<tr>
<th>${historyAccount.timestampToString(historyAccount.createdAt)}</th>
<th>${historyAccount.sender}</th>
<th>${historyAccount.receiver}</th>
<th>${historyAccount.formatKoreanWon(historyAccount.amount)}</th>
<th>${historyAccount.formatKoreanWon(historyAccount.balance)}</th>
</tr>
</c:forEach>
</tbody>
</table>
</div>
</div>
<!-- end of content.jsp(xxx.jsp) -->
</div>
</div>
<!-- footer.jsp -->
<%@ include file="/WEB-INF/view/layout/footer.jsp"%>
list.jsp
더보기
닫기
list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!-- header.jsp -->
<%@ include file="/WEB-INF/view/layout/header.jsp"%>
<!-- start of content.jsp(xxx.jsp) -->
<div class="col-sm-8">
<h2>계좌목록(인증)</h2>
<h5>Bank App에 오신걸 환영합니다.</h5>
<%-- 주소 설계 : http://localhost:8080/user/sign-up --%>
<%-- 계좌가 없는 경우와 계좌가 있는 경우를 분리할 것이다. --%>
<%-- 계좌가 있는 사용자 일 경우 반복문을 활용할 예정 --%>
<c:choose>
<c:when test="${accountList != null}">
<%-- 계좌가 존재한다. html 주석사용하면 오류 발생 : 자바코드로 변경되면 오류발생 --%>
<%-- 계좌 존재 : HTML 주석을 사용하면 오류 발생 (jstl 태그 안에서) --%>
<table class="table">
<thead>
<tr>
<th>계좌 번호</th>
<th>잔액</th>
</tr>
</thead>
<tbody>
<c:forEach var="account" items="${accountList}">
<tr>
<%-- 동적 작동하는 부분 Type=all --%>
<td> <a href="/account/detail/${account.id}?type=all">${account.number}</a> </td>
<td>${ account.formatKoreanWon(account.balance)}</td>
</tr>
</c:forEach>
</tbody>
</table>
</c:when>
<c:otherwise>
<div class="jumbotron display-4">
<h5>아직 생성된 계좌가 없습니다.</h5>
</div>
</c:otherwise>
</c:choose>
</div>
<!-- end of content.jsp(xxx.jsp) -->
</div>
</div>
<!-- footer.jsp -->
<%@ include file="/WEB-INF/view/layout/footer.jsp"%>
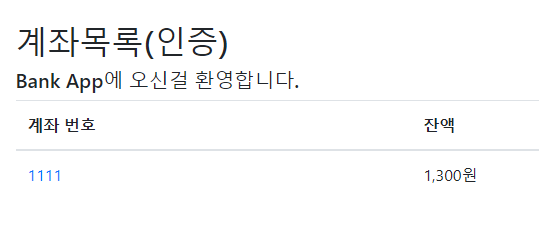
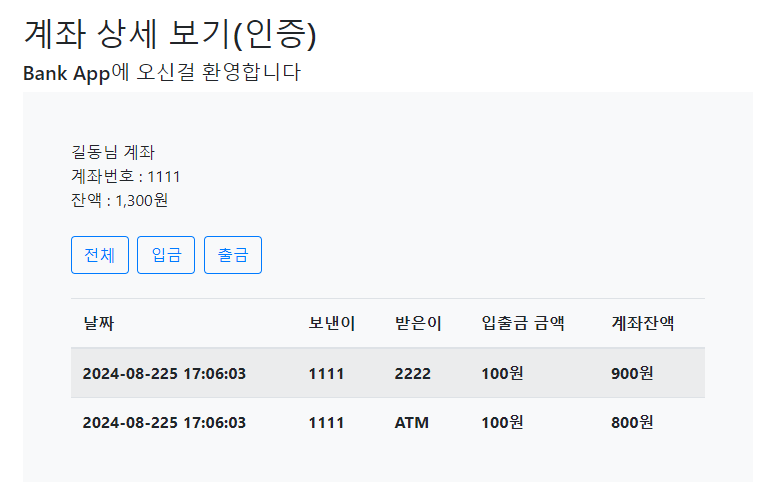